When using text files in a programm the most common use case is to read it line by line. The Java library provides the BufferedReader for this use case.
Learn by this code example how to use the BufferedReader for reading a text file.
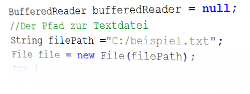
Example Code
BufferedReader bufferedReader = null;
//The path to your text file
String filePath ="C:/example.txt";
File file = new File(filePath);
try {
//BufferedReader needs a FileReader.
//You can create this while constructing
bufferedReader = new BufferedReader(new FileReader(file));
String line;
//returns null if EOF (End of File) or Error
while (null != (line = bufferedReader.readLine())) {
//Print line on the console
System.out.println(line);
//Do your work here ....
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (null != bufferedReader) {
try {
bufferedReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
The above code prints out C:\example.txt
to the console.
With bufferedReade.readLine()
one line is read from the file and returned as String. null
is returned if EOF
(End of File) is reached or an error occurs.
Be aware to catch the I/O-Exceptions properly. You should not ignore them or leave them out when working with files.
bufferedReader.close()
closes the bufferedReader. This should be done in the finally clause to ensure it is also executed if an error (Exception) occurs. It can happen that bufferedReader
never got instantiated when you try it to close in the finally
clause. Therefore you need to check for null
before closing it to avoid a NullPointerException
in your finally clause.
You will need these imports in your Java program from java.io
:
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;