Passwords are essential for interactive websites. However, passwords should never be stored unencrypted, as this represents a major security risk. In addition to other safeguards, the md5() function integrated in PHP can help here. With this you can easily determine the MD5 hash value of a string.
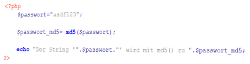
Hashing Strings with md5()
Calling md5() is self-explanatory: You pass the string whose MD5 hash value you want and you get the corresponding return string.
<?php
$password="asdf123";
$password_md5= md5($password);
echo "The string '".$password."' becomes the following with md5(): ".$password_md5;
?>
Note: Note that ‘asdf123’ is NOT a secure password and is used here for demonstration purposes only! The code results in the following output:
The string ‘asdf123’ becomes the following with md5(): 6572bdaff799084b973320f43f09b363Further parameters
Since PHP 5, the md5() function has an additional, optional parameter that can also be used to display the output in binary. To do this, you simply have to pass True as the second parameter. The default value of the parameter is False, resulting in hexadecimal output.
<?php
$password="asdf123";
$password_md5hex= md5($password);
$password_md5bin= md5($password, true);
echo "The string '".$password."' becomes with md5() :".$password_md5hex." (hex) or ".$password_md5bin." (bin)";
?>
The output of this code example is accordingly:
The string ‘asdf123’ becomes with md5() :6572bdaff799084b973320f43f09b363 (hex) or er½¯÷™K—3 ô? ³c (bin)Check encrypted passwords
MD5 is a great way to encrypt passwords. So far so good. But how can you now check whether a user has entered the correct password? After all, the MD5 hash cannot simply be decrypted again. Here’s how to get around it: You also encrypt the entered password with MD5 and check whether the hash values of the saved password and the entered one are the same. If yes, the correct password was entered. Here as a code example:
<?php
$password="asdf123";
$passwordhash= "6572bdaff799084b973320f43f09b363"; ##from database or file...
$password_md5= md5($password);
echo "<b>Entered password:</b> ".$password."<br>";
if(md5($password) == $passwordhash)
{
echo "The MD5-Hashvalues are equal. Password correct.";
}
else
{
echo "Wrong password!";
}
?>
The code produces the following output:
Entered password: asdf123The MD5-Hashvalues are equal. Password correct.
Note: The hash value of ‘asdf123’ has been hard-coded here. As a rule, however, it comes from the database or a file and must of course be requested dynamically.